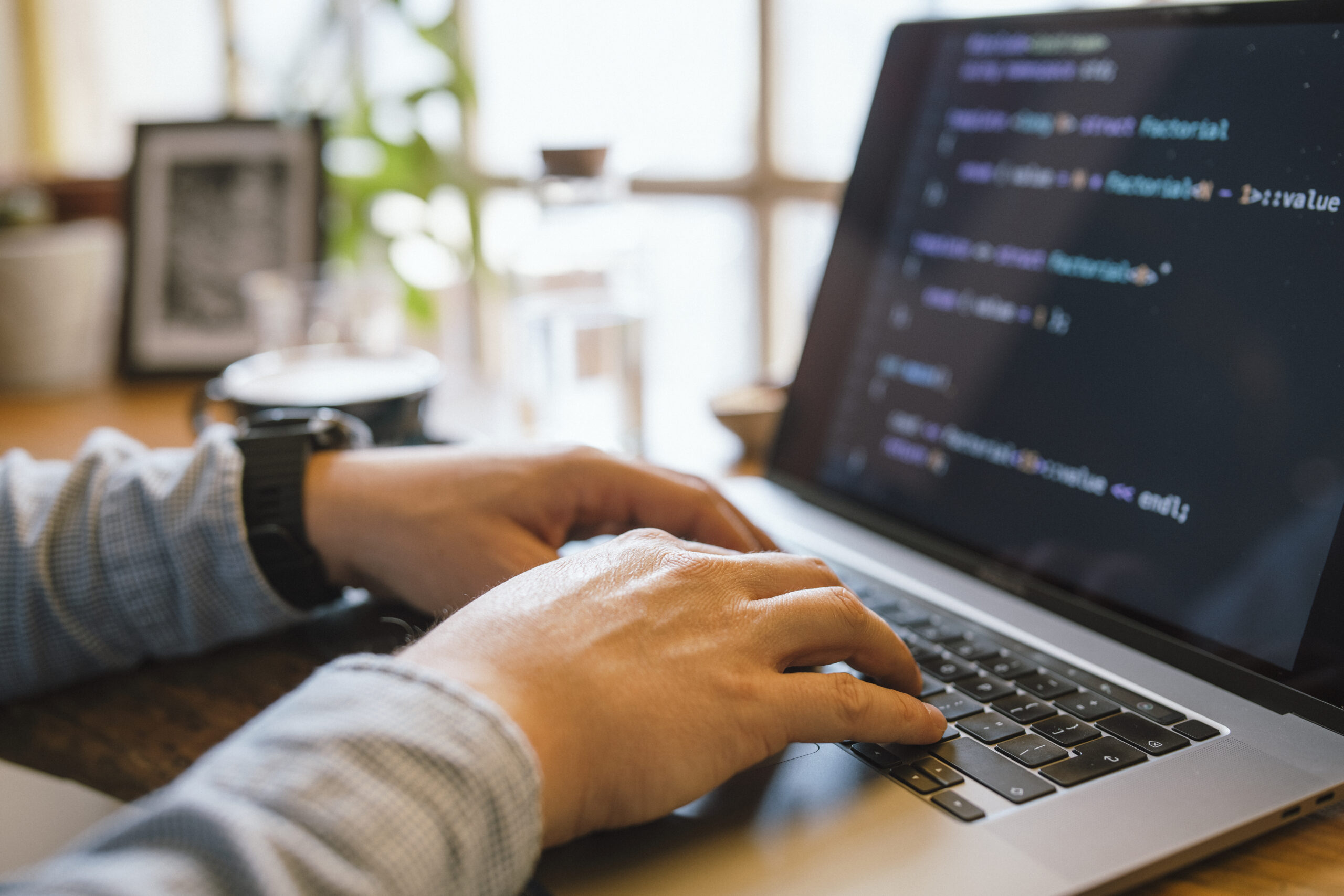
Debugging is Among the most important — nevertheless normally overlooked — expertise in the developer’s toolkit. It's actually not pretty much correcting damaged code; it’s about understanding how and why issues go Improper, and Understanding to Consider methodically to resolve troubles proficiently. No matter if you are a rookie or possibly a seasoned developer, sharpening your debugging techniques can help save hrs of irritation and dramatically improve your productivity. Here are several strategies to help builders stage up their debugging match by me, Gustavo Woltmann.
Grasp Your Resources
One of many quickest methods builders can elevate their debugging skills is by mastering the tools they use everyday. Though producing code is one particular Portion of advancement, understanding how you can connect with it correctly through execution is equally essential. Fashionable progress environments arrive equipped with impressive debugging abilities — but numerous builders only scratch the surface area of what these tools can do.
Take, one example is, an Integrated Enhancement Natural environment (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These applications enable you to set breakpoints, inspect the worth of variables at runtime, stage via code line by line, and also modify code about the fly. When applied accurately, they Allow you to notice just how your code behaves during execution, and that is priceless for monitoring down elusive bugs.
Browser developer resources, which include Chrome DevTools, are indispensable for front-conclusion developers. They assist you to inspect the DOM, watch network requests, look at genuine-time general performance metrics, and debug JavaScript inside the browser. Mastering the console, resources, and community tabs can switch disheartening UI troubles into workable duties.
For backend or process-level builders, tools like GDB (GNU Debugger), Valgrind, or LLDB supply deep Command more than managing procedures and memory management. Understanding these instruments may have a steeper Studying curve but pays off when debugging effectiveness issues, memory leaks, or segmentation faults.
Past your IDE or debugger, turn into snug with version Manage techniques like Git to be aware of code record, discover the exact second bugs have been released, and isolate problematic variations.
Ultimately, mastering your tools usually means likely beyond default settings and shortcuts — it’s about creating an intimate knowledge of your advancement surroundings to ensure when difficulties occur, you’re not missing at the hours of darkness. The greater you are aware of your applications, the greater time you may shell out resolving the particular dilemma as an alternative to fumbling by way of the method.
Reproduce the challenge
The most vital — and often ignored — steps in effective debugging is reproducing the condition. Right before leaping to the code or producing guesses, developers have to have to produce a regular surroundings or scenario where by the bug reliably seems. Without having reproducibility, fixing a bug results in being a video game of possibility, usually leading to squandered time and fragile code adjustments.
The first step in reproducing a challenge is collecting just as much context as is possible. Inquire thoughts like: What steps led to The difficulty? Which surroundings was it in — improvement, staging, or output? Are there any logs, screenshots, or error messages? The greater depth you have, the much easier it turns into to isolate the precise situations less than which the bug happens.
Once you’ve gathered adequate information, try and recreate the issue in your neighborhood atmosphere. This might mean inputting the exact same data, simulating very similar user interactions, or mimicking technique states. If The difficulty appears intermittently, look at writing automatic checks that replicate the edge situations or point out transitions concerned. These assessments don't just aid expose the situation but additionally avert regressions Down the road.
Occasionally, The problem may very well be atmosphere-distinct — it'd happen only on specific running units, browsers, or below distinct configurations. Working with tools like virtual machines, containerization (e.g., Docker), or cross-browser screening platforms is often instrumental in replicating these types of bugs.
Reproducing the issue isn’t only a phase — it’s a way of thinking. It necessitates patience, observation, along with a methodical strategy. But as soon as you can continually recreate the bug, you might be already halfway to fixing it. With a reproducible scenario, You should use your debugging equipment a lot more properly, take a look at likely fixes safely and securely, and converse additional Plainly with the staff or people. It turns an summary grievance into a concrete challenge — and that’s where builders thrive.
Read and Understand the Mistake Messages
Mistake messages are sometimes the most useful clues a developer has when anything goes Mistaken. As opposed to viewing them as irritating interruptions, builders really should study to deal with error messages as immediate communications with the technique. They usually tell you exactly what transpired, the place it occurred, and sometimes even why it transpired — if you understand how to interpret them.
Commence by reading the information meticulously and in whole. A lot of developers, specially when beneath time pressure, look at the initial line and immediately start out producing assumptions. But further while in the error stack or logs may well lie the correct root cause. Don’t just duplicate and paste error messages into search engines like google and yahoo — read through and comprehend them initially.
Break the mistake down into components. Can it be a syntax error, a runtime exception, or maybe a logic error? Does it issue to a certain file and line number? What module or operate triggered it? These inquiries can guide your investigation and position you towards the accountable code.
It’s also handy to know the terminology with the programming language or framework you’re utilizing. Error messages in languages like Python, JavaScript, or Java normally stick to predictable styles, and learning to recognize these can considerably speed up your debugging approach.
Some faults are vague or generic, As well as in those situations, it’s very important to examine the context during which the mistake happened. Verify relevant log entries, enter values, and up to date modifications while in the codebase.
Don’t forget compiler or linter warnings possibly. These frequently precede more substantial difficulties and supply hints about possible bugs.
In the end, error messages are certainly not your enemies—they’re your guides. Discovering to interpret them correctly turns chaos into clarity, aiding you pinpoint troubles speedier, cut down debugging time, and turn into a more efficient and assured developer.
Use Logging Properly
Logging is Just about the most strong instruments inside of a developer’s debugging toolkit. When made use of effectively, it provides real-time insights into how an software behaves, helping you understand what’s happening under the hood without having to pause execution or move in the code line by line.
A good logging system starts off with recognizing what to log and at what amount. Prevalent logging degrees include things like DEBUG, Details, WARN, ERROR, and FATAL. Use DEBUG for in-depth diagnostic information and facts all through enhancement, Details for standard activities (like productive begin-ups), Alert for probable troubles that don’t split the application, Mistake for true difficulties, and FATAL in the event the process can’t keep on.
Steer clear of flooding your logs with excessive or irrelevant facts. Excessive logging can obscure crucial messages and decelerate your process. Give attention to important situations, condition modifications, enter/output values, and demanding decision points as part of your code.
Format your log messages Evidently and constantly. Include context, for instance timestamps, request IDs, and performance names, so it’s easier to trace challenges in distributed units or multi-threaded environments. Structured logging (e.g., JSON logs) might make it even easier to parse and filter logs programmatically.
For the duration of debugging, logs let you observe how variables evolve, what circumstances are fulfilled, and what branches of logic are executed—all with out halting This system. They’re Particularly precious in manufacturing environments wherever stepping via code isn’t doable.
In addition, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that support log rotation, filtering, and integration with monitoring dashboards.
Finally, sensible logging is about harmony and clarity. With a effectively-considered-out logging method, it is possible to lessen the time it will take to identify challenges, acquire deeper visibility into your apps, and Increase the General maintainability and dependability within your code.
Think Just like a Detective
Debugging is not simply a technological task—it's a kind of investigation. To proficiently identify and resolve bugs, developers ought to solution the process like a detective solving a thriller. This frame of mind allows break down intricate difficulties into manageable pieces and adhere to clues logically to uncover the basis lead to.
Get started by gathering evidence. Consider the signs or symptoms of the condition: mistake messages, incorrect output, or performance concerns. Similar to a detective surveys a criminal offense scene, acquire just as much applicable information as you can without having leaping to conclusions. Use logs, test cases, and person experiences to piece together a transparent photograph of what’s happening.
Next, form hypotheses. Talk to you: What can be producing this habits? Have any alterations just lately been manufactured for the codebase? Has this concern occurred before less than identical situation? The target is usually to narrow down choices and identify opportunity culprits.
Then, take a look at your theories systematically. Try and recreate the trouble inside a managed setting. Should you suspect a specific functionality or part, isolate it and verify if The difficulty persists. Just like a detective conducting interviews, ask your code issues and Allow the results direct you closer to the reality.
Spend shut consideration to little facts. Bugs usually disguise while in the least predicted locations—just like a missing semicolon, an off-by-one particular error, or possibly a race situation. Be extensive and affected person, resisting the urge to patch The difficulty with out thoroughly knowing it. Non permanent fixes could disguise the real dilemma, only for it to resurface later on.
And lastly, maintain notes on That which you tried and uncovered. Equally as detectives log their investigations, documenting your debugging procedure can help save time for future concerns and assistance Other people fully grasp your reasoning.
By thinking like a detective, builders can sharpen their analytical competencies, method troubles methodically, and come to be more effective at uncovering hidden troubles in advanced systems.
Compose Assessments
Producing checks is one of the most effective strategies to transform your debugging skills and General advancement effectiveness. Assessments not simply assistance capture bugs early but also serve as a safety net that gives you self-assurance when generating improvements on your codebase. A effectively-examined application is easier to debug since it permits you to pinpoint specifically the place and when a challenge happens.
Begin with unit exams, which give attention to personal features or modules. These tiny, isolated exams can rapidly reveal whether or not a specific bit of logic is Doing the job as envisioned. Every time a take a look at fails, you quickly know the place to search, substantially decreasing the time used debugging. Device exams are Specifically helpful for catching regression bugs—issues that reappear just after Earlier currently being set.
Subsequent, combine integration assessments and stop-to-finish checks into your workflow. These enable be certain that numerous aspects of your software perform together effortlessly. They’re notably helpful for catching bugs that manifest in intricate methods with multiple parts or solutions interacting. If a little something breaks, your assessments can tell you which Component of the pipeline failed and less than what problems.
Writing assessments also forces you to Consider critically about your code. To check a feature adequately, you will need to be familiar with its inputs, anticipated outputs, and edge conditions. This amount of understanding In a natural way leads to higher code composition and fewer bugs.
When debugging a concern, writing a failing examination that reproduces the bug is usually a powerful initial step. As soon as the check fails continually, you are able to target correcting the bug and view your take a look at go when the issue is settled. This tactic ensures that the identical bug doesn’t return Sooner or later.
In short, creating assessments turns debugging from the frustrating guessing recreation into a structured and predictable course of action—helping you catch a lot more bugs, speedier and more reliably.
Consider Breaks
When debugging a difficult situation, it’s uncomplicated to be immersed in the problem—looking at your display for hrs, hoping Alternative after Answer. But Just about the most underrated debugging equipment is solely stepping absent. Having breaks allows you reset your mind, reduce aggravation, and often see the issue from a new perspective.
When you're too close to the code for too long, cognitive fatigue sets in. You might start overlooking obvious errors or misreading code that you wrote just several hours before. With this point out, your brain turns into significantly less effective at issue-solving. A brief wander, a coffee break, or even switching to a different endeavor for ten–15 minutes can refresh your concentrate. Many builders report acquiring the basis of an issue after they've taken the perfect time to disconnect, allowing their subconscious perform within the history.
Breaks also enable avert burnout, Specifically throughout for a longer period debugging periods. Sitting before a display, mentally stuck, is not simply unproductive but in addition draining. Stepping away means that you can return with renewed Strength along with a clearer mentality. You would possibly abruptly notice a lacking semicolon, a logic flaw, or perhaps a misplaced variable that eluded you right before.
In case you’re stuck, a superb rule of thumb will be to set a timer—debug actively for forty five–60 minutes, then take a five–10 moment break. Use that point to move all around, stretch, or do anything unrelated to code. It may come to feel counterintuitive, Particularly underneath tight deadlines, nonetheless it actually brings about faster and simpler debugging Ultimately.
In a nutshell, having breaks isn't an indication of weak spot—it’s a smart approach. It presents your brain Room to breathe, increases your perspective, and will help you steer clear of the tunnel vision That usually blocks your development. Debugging is usually a mental puzzle, and rest is a component of resolving it.
Discover From Every single Bug
Every bug you experience is much more than simply A short lived setback—It is a chance to improve to be a developer. Whether or not it’s a syntax error, a logic flaw, or maybe a deep architectural difficulty, each one can educate you one thing precious for those who make an effort to mirror and examine check here what went Erroneous.
Get started by inquiring yourself a couple of crucial inquiries when the bug is solved: What brought about it? Why did it go unnoticed? Could it are actually caught before with better practices like unit testing, code reviews, or logging? The answers often reveal blind places in the workflow or understanding and help you Create more robust coding practices relocating forward.
Documenting bugs may also be a superb behavior. Maintain a developer journal or preserve a log where you Take note down bugs you’ve encountered, the way you solved them, and Whatever you uncovered. With time, you’ll start to see patterns—recurring challenges or prevalent faults—which you could proactively keep away from.
In group environments, sharing what you've learned from the bug using your peers can be In particular highly effective. No matter whether it’s through a Slack information, a brief create-up, or A fast expertise-sharing session, aiding Other people steer clear of the very same problem boosts workforce effectiveness and cultivates a stronger Mastering tradition.
More importantly, viewing bugs as classes shifts your state of mind from irritation to curiosity. As an alternative to dreading bugs, you’ll begin appreciating them as critical areas of your development journey. In spite of everything, a number of the most effective developers are usually not the ones who generate excellent code, but individuals that continually master from their problems.
Eventually, Each and every bug you take care of adds a different layer for your ability established. So subsequent time you squash a bug, have a instant to reflect—you’ll appear absent a smarter, much more able developer thanks to it.
Conclusion
Increasing your debugging skills will take time, exercise, and patience — nevertheless the payoff is large. It makes you a more productive, self-assured, and capable developer. The following time you happen to be knee-deep inside of a mysterious bug, remember: debugging isn’t a chore — it’s a chance to become superior at what you do.